Mastering Shell Scripts: Tips and Tricks for Efficient Command Line Usage
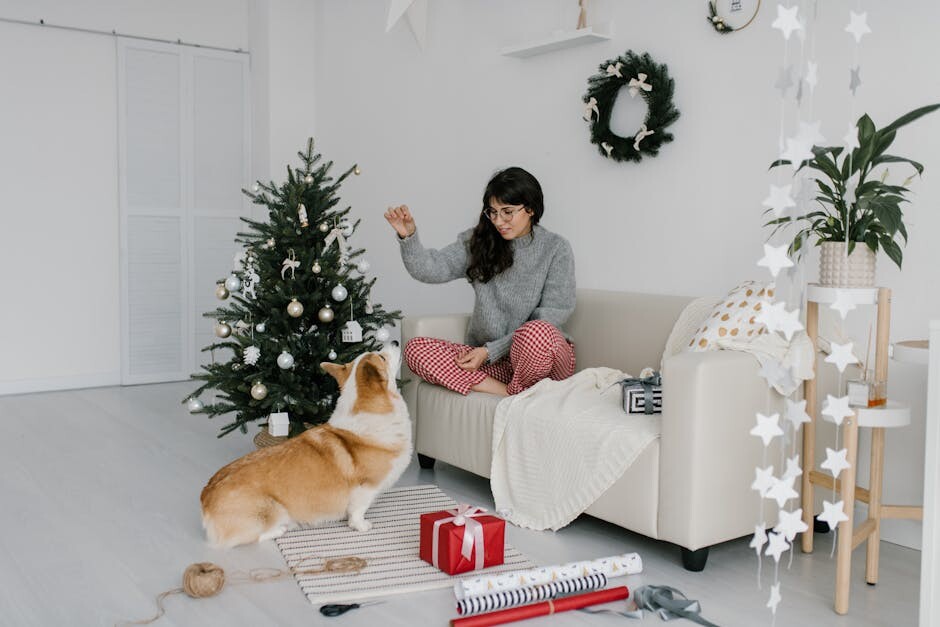
Understanding the Basics of Shell Scripts: A Comprehensive Introduction
Shell scripts are a fundamental aspect of the Unix and Linux operating systems. They provide the ability to automate repetitive tasks and improve productivity, making them a powerful tool for any system administrator or developer. This section will delve into the basics of shell scripts, giving you a comprehensive introduction to this critical subject.A shell script is a text file that contains a sequence of commands for a Unix-based operating system. These scripts are essentially a series of commands that you would normally run on your command line interface, bundled together in a single file. The shell reads this file and carries out the commands as they are listed from top to bottom.
Shell scripts use a special interpreter program, known as the shell. There are multiple shells available, each with their own distinct features, but the most commonly used shells are the Bourne shell (sh), the Bourne Again shell (bash), and the C shell (csh). The shell interprets the commands in the script, passing them on to the operating system to be executed.
Syntax is a crucial aspect of shell scripting. It's important to understand that a simple syntax error, such as a missing semicolon or incorrect spacing, can cause a shell script to fail. The basic syntax consists of commands followed by options and arguments. Commands are case-sensitive, and they are followed by any number of options (preceded by a dash) and arguments.
Variables are an important part of shell scripts, as they allow a user to store data that can be used by the script. Variables can be set using the equal sign (=) and can be accessed by using the dollar sign ($). For example, if you have a variable named ‘file’, you could assign a value to it as follows: file=example.txt. You could then access the value of this variable with $file.
Control structures, such as conditional statements and loops, are also a key part of shell scripts. Conditional statements allow the script to make decisions based on certain conditions, while loops allow a set of commands to be executed repeatedly. For example, the 'if' statement checks if a certain condition is true and then executes a set of commands if it is.
In addition to these, shell scripts often include functions. Functions are a set of instructions that perform a specific task. They help in breaking down complex scripts into smaller, more manageable parts. They can be defined using the function keyword or with parentheses and can be called by their name.
Finally, debugging is an essential part of shell scripting. Bash provides
Essential Techniques for Writing Effective Shell Scripts
Shell scripting, a powerful tool in the Unix and Linux world, enables you to automate routine tasks, customize your computing environment, and perform complex tasks more efficiently. In this section, we'll delve into some essential techniques for writing effective shell scripts.Firstly, it's crucial to understand the importance of planning your script. Before you start writing, clearly identify your script's purpose, the input it requires, and the output you expect. This clear roadmap will guide your coding process and help you avoid unnecessary work.
Next, you need to consider script portability. If you want your script to work on different Unix or Linux distributions, avoid using system-specific features. Instead, stick to generic shell commands and syntax that work across all systems. You can also specify the interpreter in the first line of your script (e.g., #!/bin/bash) to ensure the script runs on the intended shell.
Another essential technique is the use of variables. Variables allow you to store and manipulate data within your script. Always give meaningful names to your variables, which will make your script more readable. Besides, remember to quote your variables to prevent word splitting and pathname expansion.
Error handling is a critical aspect of script writing. Make sure to include checks for potential errors and handle them appropriately. By doing so, you won't leave your script users baffled by cryptic error messages. The 'set -e' command is a good practice as it makes the script exit if any command fails.
Using functions is a great way to organize your script and make it more readable. Functions allow you to encapsulate a piece of code that performs a specific task. This way, you can reuse this code whenever necessary, thus making your script more efficient and maintainable.
Comments are often overlooked, but they are essential for maintaining and enhancing your scripts. A good comment explains why something is done, not just what is being done. This makes it easier for others (or even you at a later date) to understand and modify your script.
Lastly, testing your script thoroughly is a must. Check for syntax errors, run your script with different inputs, and verify the output. Also, consider edge cases that may cause your script to fail. The more thoroughly you test your script, the more reliable it will be.
In summary, effective shell script writing involves careful planning, script portability, efficient use of variables, proper error handling, use of functions, meaningful comments, and thorough testing. By mastering these techniques, you can
Advanced Shell Scripting: Command Line Usage for Maximum Efficiency
Shell scripting is a powerful tool that can significantly enhance your productivity and efficiency when working with Unix/Linux systems. This article delves into advanced shell scripting techniques that, when mastered, can streamline your command line usage and boost your productivity.The first technique to consider is the use of command-line arguments. These are the inputs that you provide to your scripts when you run them. For example, in the command `./myscript.sh arg1 arg2`, `arg1` and `arg2` are command-line arguments. They can be accessed in your script using the variables `$1`, `$2`, etc. This feature is particularly useful when you need to create scripts that are flexible and can be customized each time they are run.
Next is the concept of exit statuses. Every command that you run in the shell provides an exit status, a numeric value that indicates whether the command succeeded or failed. By convention, an exit status of 0 means success, while any other value signifies failure. You can access the exit status of the last command using the special variable `$?`. This is beneficial for error handling in your scripts.
Another advanced technique is the use of here documents. A here document is a type of redirection that allows you to feed a command sequence to an interactive program or a command, such as ftp, cat, or ssh. It's defined with the `<<` operator followed by a delimiter. The shell interprets the lines following this operator until it encounters the delimiter as a single command.
Next up is the use of functions in your scripts. Functions are a way to group commands for later execution, improving the readability and maintainability of your scripts. They work similarly to functions in other programming languages. You can declare a function with the `function` keyword or just by using the function name followed by parentheses.
Lastly, consider the use of control structures. These include if-else statements, for and while loops, and case statements. If-else statements are used for decision making, loops are used for repeating tasks, and case statements are used for multi-way branching. Mastering these control structures can greatly increase your scripts' flexibility and power.
In addition to these techniques, there are several best practices that you should follow when writing shell scripts. For instance, always use double quotes around variables to prevent word splitting and globbing. Also, use the `[[` command for tests instead of `[` because it's more powerful and less error-prone.
In conclusion, advanced shell scripting
Troubleshooting Common Issues in Shell Scripting: Practical Solutions
Shell scripting is a potent tool, allowing us to automate tasks, manage files, and efficiently handle data. Whether you're a system administrator or a developer, mastering shell scripts can make your work easier and more efficient. However, it is not uncommon to encounter some issues while writing and executing shell scripts. Let's delve into some of the common problems you might face and how to troubleshoot them.One common issue encountered in shell scripting is syntax errors. These can occur due to misspelled commands, incorrect use of special characters, or wrong indentation. Paying attention to the error messages displayed in the console can help you identify the line of code causing the error. Most shell interpreters provide descriptive error messages that can guide you towards the problem. Moreover, using a code editor that highlights syntax can prevent many of these issues.
Another frequent problem is incorrect variable usage. Variables in shell scripts are sensitive to spaces. For instance, writing VAR = 1 instead of VAR=1 will result in an error. Similarly, referencing a variable requires a preceding dollar sign ($), like $VAR. Failing to do this will cause the script to treat the variable as a string. Always double-check your variable declarations and references to avoid such issues.
Permission issues can also prevent your script from executing correctly. If you're running a script that requires administrative privileges without the necessary permissions, it will fail. The solution is to either run the script as a user with the required permissions or modify the permissions of the script file using the chmod command.
Paths can also be a source of trouble in shell scripting. Absolute paths are specific and unchanging, but they can cause problems if the script is run on a different machine or if files are moved. On the other hand, relative paths are more flexible but can lead to issues if the script is run from a different directory. To prevent path issues, use the dirname command to get the script's directory and build paths from there.
Globbing and regex issues frequently occur in shell scripts. The shell might interpret characters like asterisks (*) or question marks (?) as wildcard characters, leading to unexpected results. To prevent this, enclose these characters in quotes to force the shell to treat them as literal characters.
Lastly, always keep in mind the portability of your script. If you're writing a script that will be used across different systems or different shell interpreters, make sure to stick to standard syntax and avoid using features specific to a certain shell unless absolutely necessary.
Troub
Streamlining Your Workflow: Time-Saving Tips and Tricks in Shell Scripting
Shell scripting is an invaluable skill to possess. It allows you to automate tasks, saving you time and effort, and enables you to perform complex operations with just a few lines of code. Here are some tips and tricks to streamline your workflow and make your shell scripts more efficient.1. Use Variables: Variables can be a great way to make your shell scripts more flexible. Instead of hardcoding values into your script, use variables so that the values can be changed easily without modifying the script itself. This is particularly useful when you need to use the same value multiple times throughout your script.
2. Comment Your Code: This might seem like an unnecessary step, especially if you're the only one who will be using your script. However, commenting your code is a good habit to get into. It makes it easier for you (or someone else) to understand what the script is doing if you need to modify or debug it later.
3. Use Functions: Functions can help to make your shell scripts more modular and easier to read. Each function should perform a specific task and can be called multiple times throughout your script. This can also help to reduce code duplication.
4. Check for Errors: Always check the exit status of your commands. This will allow your script to handle errors gracefully and prevent it from continuing with incorrect or unexpected data. You can use the $? variable to get the exit status of the last command.
5. Use Command Line Arguments: Command line arguments can make your shell scripts more versatile. They allow you to pass different inputs to your script each time you run it. This can be particularly useful for scripts that perform tasks on different files or directories.
6. Use Conditional Statements: Conditional statements allow your script to make decisions based on certain conditions. For example, you might want your script to perform a different action depending on whether a file exists or not.
7. Use Loops: Loops can be used to perform repetitive tasks. For example, you might want to process all files in a directory or perform an action a certain number of times.
8. Test Your Scripts: Always test your scripts thoroughly before using them in a production environment. Try to anticipate all possible scenarios and make sure your script handles them correctly.
9. Keep Learning: There are many resources available online to help you improve your shell scripting skills. Keep learning and experimenting with new techniques to improve your efficiency and productivity.
10. Use a Version Control System: This might seem overkill for small scripts,