10 Essential JavaScript Functions Every Developer Should Know
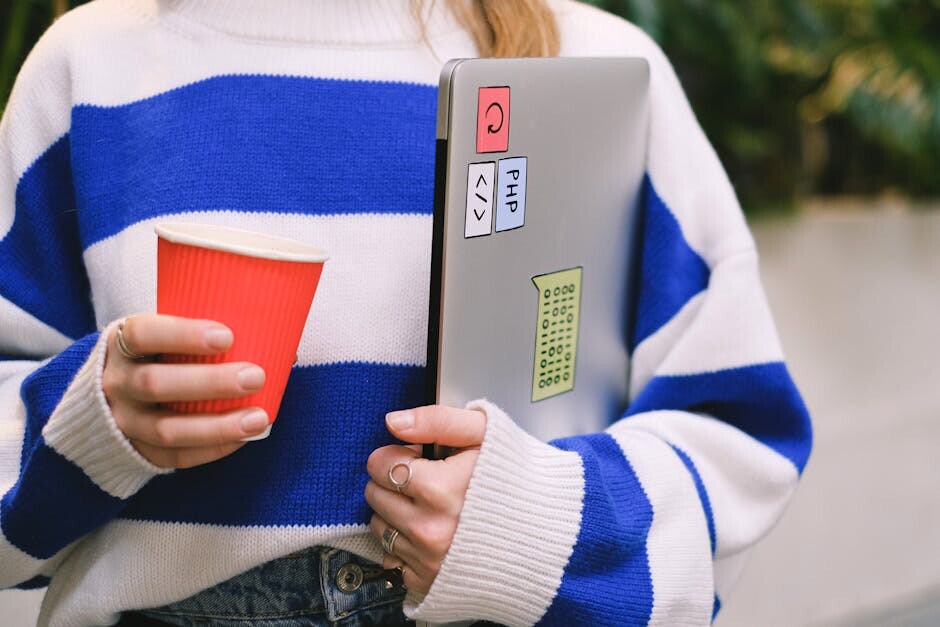
Understanding the Core of JavaScript: Essential Functions
JavaScript is renowned as the backbone of web development, powering everything from simple animations to complex web applications. A deep understanding of its core functions is crucial for every developer. It's not just about knowing the syntax but understanding how and when to use these functions to build more robust, efficient, and maintainable code. In this section, we're going to dive into ten essential JavaScript functions that every developer should know.1. Array.prototype.map()
The map() function is a high-order function that transforms each element in an array based on a callback function. It doesn't modify the original array but instead creates a new one with the transformed elements. This function is handy for transforming data.
2. Array.prototype.filter()
The filter() function is another high-order function that creates a new array of elements that pass a specified condition. This function is ideal for situations where you want to remove certain elements from an array.
3. Array.prototype.reduce()
The reduce() function is a bit more complex but incredibly powerful. It applies a function against an accumulator and each element in the array (from left to right) to reduce it to a single value. This function is often used to compute a single value from an array.
4. JSON.parse() and JSON.stringify()
JSON.parse() and JSON.stringify() are used for working with JSON data. JSON.parse() converts a JSON string into a JavaScript object, while JSON.stringify() does the opposite, converting a JavaScript object into a JSON string.
5. console.log()
Arguably the most used function, console.log() is used for debugging. It prints the output to the console, which is helpful for tracking variables and the flow of code.
6. setTimeout() and setInterval()
setTimeout() and setInterval() are built-in timing functions. setTimeout() executes a function after a specified number of milliseconds, while setInterval() keeps executing a function repeatedly after every given time-interval until it's stopped.
7. Function.prototype.bind()
The bind() function allows you to set the 'this' value now while allowing you to execute the function in the future. It's commonly used in event handlers and callbacks.
8. Object.keys()
Object.keys() is a method that returns the keys (property names) of an object. It's useful when you need to loop through an object's properties.
9. Array.prototype.sort()
The sort() function is used to sort the elements of an array in place and returns the array. By default, it sorts elements as strings, but you
Top 10 JavaScript Functions: An In-Depth Exploration
JavaScript stands at the forefront of modern web development. Its versatility and power have made it a staple for developers around the globe. Here, we'll explore the top 10 JavaScript functions that every developer should know to improve their coding prowess.1. **Array.map()**: This high-order function creates a new array by calling a provided function on every element in the calling array. It doesn't change the original array, but returns a new one, making it perfect when you need to manipulate data in an array without altering the original.
2. **Array.reduce()**: This function reduces an array to a single value by executing a provided function on each element of the array (from left to right) and the return value of the function is stored in an accumulator. The reduce() method can be incredibly handy when you need to find a cumulative or concatenated value in an array.
3. **Array.filter()**: This function creates a new array with all elements that pass a test implemented by the provided function. It's perfect for quickly creating a new array that only includes certain values.
4. **Array.forEach()**: This function executes a provided function once for each array element. It's a great alternative to traditional for loops, making your code cleaner and more readable.
5. **Function.bind()**: The bind() method creates a new function that, when called, has its this keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called. This is especially useful in maintaining context in asynchronous JavaScript.
6. **Array.sort()**: This function sorts the elements of an array in place and returns the array. The sort order can be either built-in ascending or descending, or defined by a custom sort function. It's a crucial tool when working with data that needs to be presented in a certain order.
7. **Array.find()**: This method returns the value of the first element in the array that satisfies the provided testing function. This is useful when you need to find a specific value in an array based on certain criteria.
8. **Object.keys()**: This method returns an array of a given object's own enumerable property names, iterated in the same order that a normal loop would. It's vital when you need to manipulate or access an object's keys.
9. **Array.some()**: This method tests whether at least one element in the array passes the test implemented by the provided function. It's a quick and easy way to check
The Developer's Toolkit: 10 JavaScript Functions You Need
JavaScript is a critical language for web development, and mastering its functions can significantly enhance your coding abilities. Here are ten essential JavaScript functions that every developer should be proficient with.1. **Array.prototype.map()**: This function is used to transform each element in an array without altering the original array. It takes a callback function as an argument, which it applies to every item in the array, returning a new array with the transformed items.
2. **Array.prototype.filter()**: This function is used to filter out elements from an array that do not meet certain criteria. It also takes a callback function as an argument, which should return a boolean value. The callback is applied to every item, and a new array is returned with only the items that returned true.
3. **Array.prototype.reduce()**: The reduce() function is one of the most powerful array methods in JavaScript. It's used to reduce an array to a single value - for example, to calculate the sum of all elements in an array.
4. **setTimeout() and setInterval()**: These two functions are used to control the timing of events in your code. setTimeout() executes a function after a certain amount of time has passed, while setInterval() repeats the execution of a function at specified intervals.
5. **JSON.parse() and JSON.stringify()**: JSON.parse() is used to convert a JSON string into a JavaScript object, while JSON.stringify() does the opposite – it converts a JavaScript object into a JSON string. These functions are essential for working with JSON data in JavaScript.
6. **console.log()**: This is a fundamental debugging tool in JavaScript that prints output to the console. It can be used to display values of variables, results of functions, and to identify errors in the code.
7. **querySelector() and querySelectorAll()**: These functions allow you to access DOM elements using CSS selectors. querySelector() returns the first element that matches the specified selectors, while querySelectorAll() returns all matching elements in a Node List.
8. **addEventListener()**: This function is used to handle events in JavaScript. It allows you to specify a function to be executed when a specific event occurs on a specific element.
9. **Math.random()**: This function generates a random number between 0 (inclusive) and 1 (exclusive). It's often used in games, simulations, and whenever you need to generate a random outcome.
10. **Promise()**: Promises in JavaScript represent an eventual completion or failure of an
Enhancing Your Coding Skills: Essential JavaScript Functions
JavaScript is an essential coding language for any aspiring or experienced web developer. It offers a myriad of functions that can optimize the development process, streamline coding efforts and enhance overall coding skills. This section will focus on ten essential JavaScript functions that every developer should know to enhance their abilities.1. `console.log()`: This is arguably the most frequently used JavaScript function. It's ideal for debugging and allows developers to print content to the JavaScript console. Using `console.log()`, developers can track variables, function outputs, and more, making it one of the most essential tools for developers.
2. `alert()`: This is a simple yet powerful JavaScript function used for displaying alert dialog boxes with a specified message. While it's not a best practice for user-facing interactions, it is a useful tool during the development process to quickly surface information.
3. `prompt()`: This function is used to display a dialog box that prompts the visitor for input. The `prompt()` function lets you pause the execution of code until the user responds to the input, making it a very useful tool for interactive websites.
4. `parseInt() and parseFloat()`: These two functions are used to convert string numeric values into integer and floating-point numbers, respectively. These are essential functions when dealing with operations that need numerical input.
5. `Array.map()`: This function is used to create a new array by performing a function on each element in an initial array. `Array.map()` does not change the original array, making it a pure function that's very useful in functional programming.
6. `Array.filter()`: This function is used to create a new array from an existing one, including only those elements that meet a certain condition. This is a powerful function for working with arrays and manipulating data.
7. `setTimeout() and setInterval()`: These functions allow developers to control the execution of their code based on time. `setTimeout()` executes a block of code once after a set amount of time, whereas `setInterval()` executes a block of code repeatedly, with a fixed time delay between each call.
8. `JSON.parse() and JSON.stringify()`: These functions are used to convert objects into JSON format strings and vice versa. They are crucial when dealing with JSON data, which is a common task in web development.
9. `Array.reduce()`: This function is used to reduce an array to a single value by executing a provided function for each value of the array. This is especially useful in calculations where you
Streamlining Web Development: Must-Know JavaScript Functions
JavaScript is an essential programming language for web development. It provides multiple functions that streamline the web development process, making it easier and more efficient. This article will discuss some of the most important JavaScript functions that every web developer should know.1. **Array.prototype.map()**: This JavaScript function is used to create a new array with the results of a function on every element in the calling array. It's beneficial when you want to manipulate or change all elements in an array, without altering the original one.
2. **Array.prototype.filter()**: This function creates a new array with all elements that pass a test implemented by the provided function. It's useful when you want to create a subset of an array that meets certain criteria.
3. **Array.prototype.reduce()**: The reduce() function applies a function against an accumulator and each element in the array (from left to right) to reduce it to a single value. It's often used for calculations that need to use all elements in an array.
4. **Array.prototype.forEach()**: This function executes a provided function once for each array element. It's a cleaner and more readable alternative to traditional for loops.
5. **JSON.parse() and JSON.stringify()**: These are two essential functions for working with JSON data. JSON.parse() converts a JSON string into a JavaScript object, while JSON.stringify() does the opposite - it converts a JavaScript object into a JSON string.
6. **console.log()**: Despite its simplicity, console.log() is a critical function for debugging. It prints any JavaScript value (number, string, object, etc.) to the console.
7. **setTimeout() and setInterval()**: These are timing functions. setTimeout() is used to execute a function or code after a specified delay, while setInterval() repeatedly calls a function or executes a code snippet, with a fixed time delay between each call.
8. **Promise and async/await**: Promises and async/await are used for handling asynchronous operations. A Promise is an object that may produce a single value at some point in the future. The async function returns a Promise, and the await expression is used to wait for the Promise to resolve or reject.
9. **Array.prototype.some() and Array.prototype.every()**: These functions are used to test arrays for some or every element passing a certain test. some() checks if at least one element passes the test, while every() checks if all elements pass the test.
10. **Function.prototype.bind()**: This function